How Builders Can Make improvements to Their Debugging Capabilities By Gustavo Woltmann
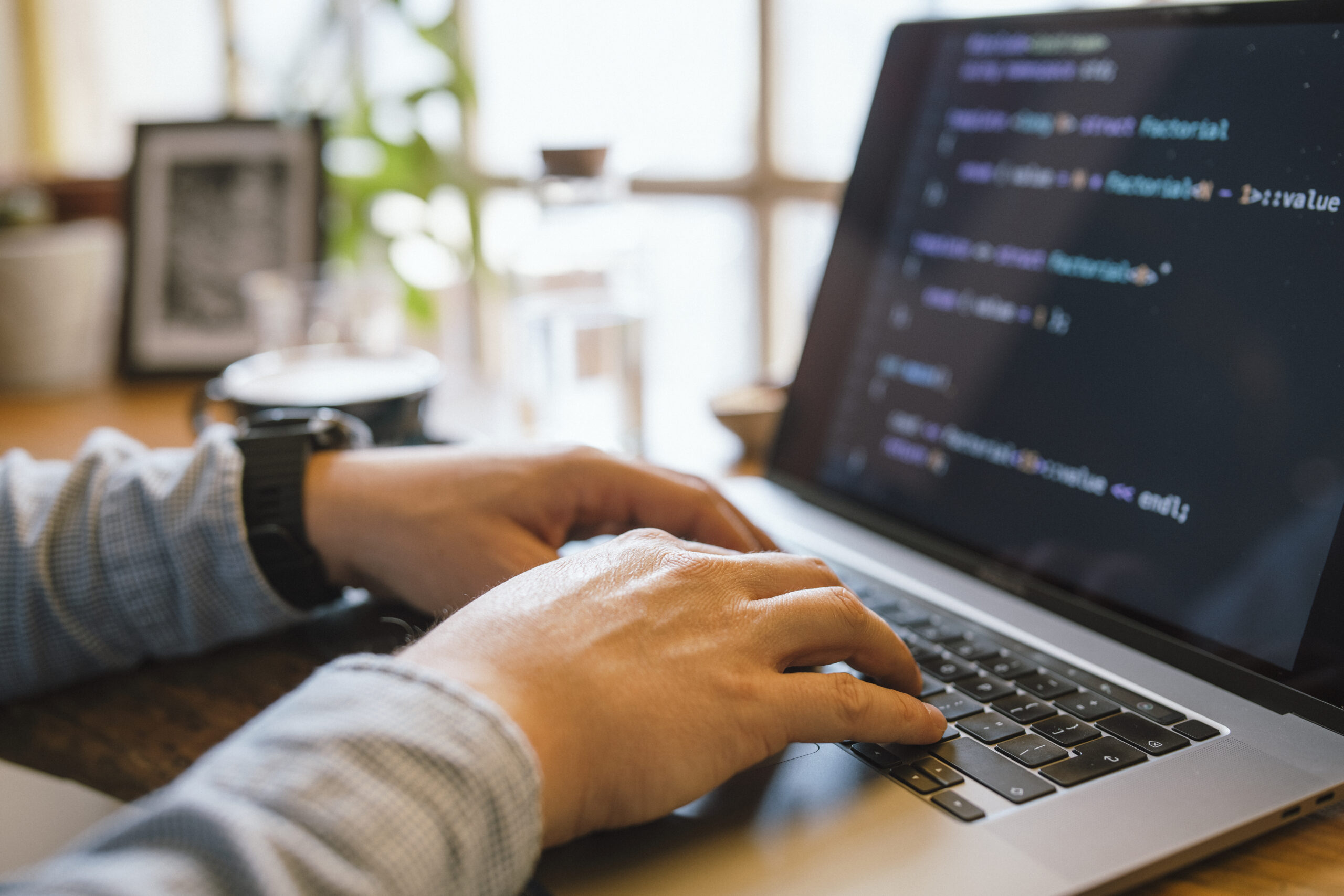
Debugging is Probably the most necessary — yet frequently neglected — techniques inside of a developer’s toolkit. It's not just about fixing damaged code; it’s about being familiar with how and why things go wrong, and Studying to Believe methodically to solve challenges successfully. Irrespective of whether you are a starter or simply a seasoned developer, sharpening your debugging competencies can save hours of frustration and dramatically improve your efficiency. Here i will discuss numerous techniques to assist developers amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Instruments
One of several quickest methods builders can elevate their debugging techniques is by mastering the instruments they use every single day. When composing code is a single part of enhancement, figuring out the way to communicate with it successfully during execution is Similarly crucial. Modern enhancement environments occur Outfitted with powerful debugging abilities — but several builders only scratch the floor of what these tools can do.
Acquire, as an example, an Built-in Growth Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These instruments enable you to set breakpoints, inspect the worth of variables at runtime, step by code line by line, and also modify code within the fly. When used accurately, they let you notice specifically how your code behaves throughout execution, that's a must have for tracking down elusive bugs.
Browser developer applications, for example Chrome DevTools, are indispensable for entrance-end builders. They permit you to inspect the DOM, watch network requests, perspective actual-time overall performance metrics, and debug JavaScript within the browser. Mastering the console, resources, and community tabs can flip frustrating UI concerns into workable jobs.
For backend or procedure-degree builders, instruments like GDB (GNU Debugger), Valgrind, or LLDB provide deep Management around working processes and memory administration. Discovering these resources could have a steeper Discovering curve but pays off when debugging general performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, develop into comfortable with Edition Handle systems like Git to comprehend code heritage, come across the precise moment bugs were introduced, and isolate problematic variations.
Ultimately, mastering your tools indicates heading over and above default options and shortcuts — it’s about establishing an intimate knowledge of your advancement surroundings to ensure when difficulties occur, you’re not lost in the dark. The better you understand your resources, the more time you are able to invest fixing the actual difficulty as opposed to fumbling by means of the process.
Reproduce the issue
Probably the most essential — and sometimes disregarded — measures in efficient debugging is reproducing the issue. Before leaping into the code or earning guesses, builders will need to make a constant atmosphere or state of affairs where the bug reliably seems. With no reproducibility, fixing a bug becomes a activity of probability, usually resulting in wasted time and fragile code variations.
Step one in reproducing an issue is accumulating just as much context as possible. Inquire questions like: What steps led to The difficulty? Which surroundings was it in — development, staging, or generation? Are there any logs, screenshots, or mistake messages? The more element you've, the a lot easier it gets to isolate the exact problems below which the bug occurs.
As soon as you’ve collected ample info, endeavor to recreate the trouble in your neighborhood natural environment. This could indicate inputting exactly the same facts, simulating comparable consumer interactions, or mimicking system states. If The difficulty appears intermittently, take into consideration creating automatic checks that replicate the edge scenarios or condition transitions included. These tests not merely assistance expose the issue and also prevent regressions Later on.
Sometimes, The problem can be environment-certain — it'd materialize only on particular functioning methods, browsers, or beneath unique configurations. Applying resources like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms may be instrumental in replicating this sort of bugs.
Reproducing the situation isn’t simply a move — it’s a state of mind. It needs endurance, observation, and a methodical method. But after you can persistently recreate the bug, you happen to be now midway to correcting it. Which has a reproducible circumstance, You may use your debugging applications more effectively, test possible fixes safely, and converse far more Plainly using your crew or end users. It turns an abstract grievance into a concrete problem — and that’s exactly where developers prosper.
Browse and Have an understanding of the Mistake Messages
Mistake messages will often be the most beneficial clues a developer has when a little something goes Erroneous. In lieu of observing them as aggravating interruptions, developers should find out to treat mistake messages as immediate communications from your method. They frequently show you just what exactly transpired, wherever it occurred, and occasionally even why it transpired — if you understand how to interpret them.
Commence by reading the information carefully As well as in total. Many builders, especially when less than time stress, look at the first line and promptly commence creating assumptions. But further inside the mistake stack or logs could lie the correct root result in. Don’t just duplicate and paste error messages into search engines like google — browse and realize them first.
Split the error down into parts. Can it be a syntax error, a runtime exception, or maybe a logic error? Does it point to a particular file and line number? What module or functionality induced it? These thoughts can guidebook your investigation and issue you toward the dependable code.
It’s also helpful to grasp the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically follow predictable designs, and Mastering to recognize these can substantially increase your debugging approach.
Some errors are vague or generic, As well as in Those people instances, it’s critical to look at the context in which the mistake happened. Verify relevant log entries, enter values, and up to date modifications while in the codebase.
Don’t ignore compiler or linter warnings possibly. These generally precede larger problems and provide hints about probable bugs.
Finally, error messages aren't your enemies—they’re your guides. Finding out to interpret them properly turns chaos into clarity, aiding you pinpoint troubles speedier, cut down debugging time, and turn into a more effective and assured developer.
Use Logging Properly
Logging is One of the more powerful tools inside a developer’s debugging toolkit. When employed efficiently, it provides actual-time insights into how an software behaves, supporting you understand what’s happening underneath the hood without having to pause execution or move in the code line by line.
A very good logging system starts off with knowing what to log and at what level. Common logging concentrations involve DEBUG, Facts, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic details throughout improvement, INFO for typical situations (like prosperous start off-ups), WARN for possible concerns that don’t break the applying, ERROR for real problems, and Lethal if the method can’t continue.
Steer clear of flooding your logs with too much or irrelevant facts. Excessive logging can obscure essential messages and decelerate your technique. Give attention to key events, point out improvements, input/output values, and important final decision points in the code.
Format your log messages Evidently and persistently. Consist of context, like timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
All through debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re Primarily useful in output environments exactly where stepping by code isn’t attainable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about stability and clarity. That has a nicely-assumed-out logging method, you may reduce the time it will take to identify challenges, acquire deeper visibility into your apps, and Increase the Total maintainability and trustworthiness of your code.
Feel Just like a Detective
Debugging is not merely a technical job—it's a sort of investigation. To correctly recognize and deal with bugs, builders must method the method just like a detective fixing a secret. This mentality helps stop working advanced issues into manageable components and stick to clues logically to uncover the basis lead to.
Start out by accumulating proof. Look at the signs and symptoms of the trouble: error messages, incorrect output, or functionality troubles. The same as a detective surveys a criminal offense scene, accumulate just as much appropriate information as you can without leaping to conclusions. Use logs, exam conditions, and person stories to piece jointly a clear image of what’s occurring.
Following, kind hypotheses. Request your self: What might be causing this actions? Have any variations not long ago been designed on the codebase? Has this challenge transpired prior to under identical situation? The purpose is always to narrow down options and discover probable culprits.
Then, examination your theories systematically. Attempt to recreate the problem in a very controlled atmosphere. For those who suspect a selected operate or component, isolate it and confirm if The problem persists. Like a detective conducting interviews, check with your code queries and let the final results lead you nearer to the truth.
Pay back near notice to compact information. Bugs frequently disguise inside the the very least anticipated sites—just like a lacking semicolon, an off-by-one particular error, or a race issue. Be thorough and individual, resisting the urge to patch the issue with no fully comprehension it. Temporary fixes may possibly disguise the real problem, only for it to resurface afterwards.
Lastly, hold notes on what you experimented with and acquired. Just as detectives log their investigations, documenting your debugging approach can help you save time for potential challenges and assist Some others understand your reasoning.
By pondering similar to a detective, builders can sharpen their analytical abilities, technique complications methodically, and become simpler at uncovering concealed problems in sophisticated devices.
Generate Tests
Creating exams is among the simplest tips on how to enhance your debugging expertise and In general development efficiency. Exams not just support capture bugs early and also function a security Internet that offers you assurance when creating adjustments to the codebase. A properly-examined software is simpler to debug since it lets you pinpoint just the place and when a challenge happens.
Get started with device assessments, which center on particular person capabilities or modules. These modest, isolated assessments can speedily reveal regardless of whether a particular piece of logic is Doing work as anticipated. Whenever a check fails, you instantly know where to glimpse, noticeably cutting down enough time invested debugging. Unit checks are especially practical for catching regression bugs—difficulties that reappear immediately after Formerly becoming fixed.
Upcoming, combine integration tests and close-to-conclusion exams into your workflow. These assist make sure that various portions of your application work alongside one another efficiently. They’re especially practical for catching bugs that arise in complicated units with a number of components or solutions interacting. If a little something breaks, your exams can tell you which Section of the pipeline failed and underneath what circumstances.
Crafting exams also forces you to definitely Feel critically regarding your code. To test a function adequately, you will need to be familiar with its inputs, anticipated outputs, and edge cases. This amount of understanding In a natural way leads to higher code composition and fewer bugs.
When debugging a problem, creating a failing take a look at that reproduces the bug can be a strong starting point. Once the examination fails continuously, you are able to center on correcting the bug and observe your take a look at go when the issue is fixed. This approach makes sure that the exact same bug doesn’t return in the future.
In brief, composing checks turns debugging from the irritating guessing match right into a structured and predictable system—assisting you catch more bugs, quicker and even more reliably.
Acquire Breaks
When debugging a tough issue, it’s simple to become immersed in the trouble—observing your display screen for several hours, seeking solution following Alternative. But one of the most underrated debugging tools is simply stepping away. Taking breaks assists you reset your thoughts, minimize disappointment, and sometimes see The problem from a new viewpoint.
When you're as well near to the code for also prolonged, cognitive tiredness sets in. You could commence overlooking clear mistakes or misreading code which you wrote just hours earlier. In this point out, your Mind gets fewer economical at challenge-fixing. A short walk, a espresso split, as well as switching to a special job for ten–quarter-hour can refresh your emphasis. Several developers report finding the root of a dilemma once they've taken time for you to disconnect, letting their subconscious do the job while in the track record.
Breaks also help reduce burnout, In particular for the duration of lengthier debugging classes. Sitting down in front of a screen, mentally trapped, is not just unproductive but also draining. Stepping absent permits you to return with renewed energy and also a clearer attitude. You might quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
When you’re stuck, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–ten minute crack. Use that time to maneuver around, extend, or do something unrelated to code. It could feel counterintuitive, especially beneath limited deadlines, nevertheless it basically results in a lot quicker and more effective debugging In the long term.
In short, using breaks is not really a sign of weak point—it’s a sensible technique. It offers your Mind Room to breathe, increases website your perspective, and will help you steer clear of the tunnel vision that often blocks your development. Debugging is usually a mental puzzle, and rest is a component of resolving it.
Learn From Each and every Bug
Just about every bug you encounter is more than simply A short lived setback—It is a chance to improve to be a developer. Whether or not it’s a syntax error, a logic flaw, or maybe a deep architectural difficulty, each one can teach you one thing worthwhile for those who take the time to reflect and evaluate what went Mistaken.
Get started by asking your self a couple of crucial inquiries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with better practices like device tests, code assessments, or logging? The responses normally expose blind spots in your workflow or understanding and assist you to Develop stronger coding habits moving ahead.
Documenting bugs will also be a wonderful pattern. Retain a developer journal or retain a log in which you Notice down bugs you’ve encountered, how you solved them, and what you learned. Eventually, you’ll start to see styles—recurring difficulties or widespread blunders—which you can proactively steer clear of.
In team environments, sharing Anything you've figured out from a bug together with your friends is often Specially effective. Whether it’s via a Slack concept, a short produce-up, or A fast information-sharing session, helping Many others stay away from the exact same difficulty boosts crew efficiency and cultivates a much better Understanding culture.
Additional importantly, viewing bugs as lessons shifts your mentality from disappointment to curiosity. Rather than dreading bugs, you’ll start out appreciating them as crucial aspects of your growth journey. In any case, some of the ideal developers will not be the ones who compose perfect code, but individuals who continuously understand from their mistakes.
Ultimately, Each individual bug you resolve provides a brand new layer on your skill set. So upcoming time you squash a bug, have a second to replicate—you’ll arrive absent a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging capabilities takes time, apply, and endurance — but the payoff is huge. It can make you a far more efficient, assured, and capable developer. The subsequent time you might be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to become far better at That which you do.